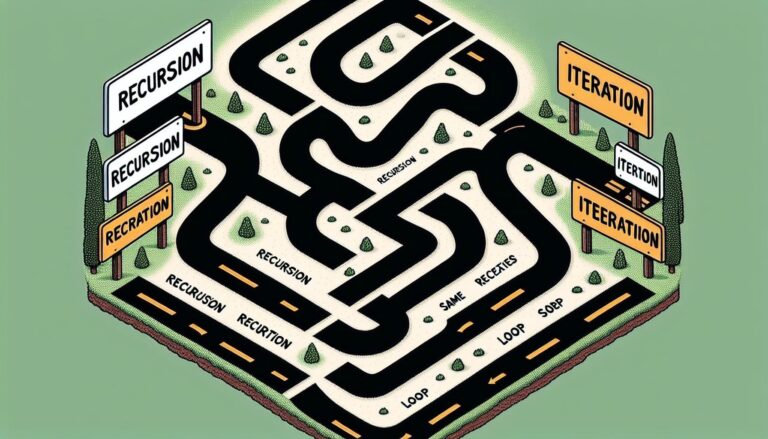
Difference Between Recursion and Iteration is a fundamental concept in computer science that explores two distinct approaches to problem-solving. Understanding these techniques helps programmers choose the most efficient method for their coding challenges, impacting performance and readability.
Understanding Recursion and Iteration in Programming
In programming, both recursion and iteration are techniques used to repeat a certain block of code, but they operate in fundamentally different ways. Recursion involves a function calling itself to solve smaller instances of the same problem until it reaches a base case. This method is highly expressive, allowing complex algorithms to be solved with elegant code, especially in data structures like trees. In contrast, iteration uses loops to repeat code until a specified condition is met, making it often more efficient in terms of memory usage, since it typically avoids the overhead of multiple function calls associated with recursion.
Understanding the Difference Between Recursion and Iteration is essential for developers. Here are some key points to consider:
- Performance: Iteration tends to consume less memory than recursion, as it operates within a single function call.
- Readability: Recursive code can be more readable when dealing with problems that are naturally recursive, like factorial calculations or tree traversals.
- Complexity: While recursion can simplify code, it may also introduce the risk of stack overflow if not controlled properly.
Aspect | Recursion | Iteration |
---|---|---|
Memory Usage | Typically higher | Lower |
Ease of Use | Can be easier for complex structures | More straightforward for simpler problems |
Control | Explicit base cases are needed | Condition-based loop control |
Ultimately, mastering the Difference Between Recursion and Iteration will enhance your problem-solving skills in programming. Each method has its unique strengths, and the right choice depends on the specific scenario you are tackling. Whether you choose recursion for its elegance or iteration for its efficiency, understanding these concepts is crucial for any aspiring programmer.
The Fundamental Concepts of Recursion
At the core of computer science lies recursion, a technique where a function calls itself to solve smaller instances of the same problem. This approach simplifies complex problems into manageable parts, often leading to more intuitive and cleaner code. Fundamental to recursion are two key components: the base case and the recursive case. The base case is crucial because it defines the condition under which the recursion will stop, preventing infinite loops. The recursive case, on the other hand, dictates how the problem is broken down into smaller subproblems. Understanding these concepts is essential to grasping the fundamental differences between recursion and iteration.
While iteration involves looping through a sequence until a condition is met, recursion eliminates the need for explicit loops by allowing functions to repeat themselves. This can lead to elegantly structured programs but may require more memory due to the function call stack. The difference between recursion and iteration can sometimes be a matter of performance and readability. Developers often prefer recursion for problems that can naturally fit its approach, such as tree traversals and certain algorithms like the calculation of factorial numbers. However, excessive use of recursion can lead to stack overflow errors, prompting engineers to evaluate which method best suits their specific application.
The Core Principles of Iteration
Iteration is fundamentally about repeating a set of instructions until a particular condition is met. This concept is rooted in the idea of progress through repetition, allowing for structures in programming that are both dynamic and efficient. By employing loops, such as for and while, iterative processes enable developers to manipulate data collections and perform tasks with reduced complexity. In contrast to recursion, which may introduce layers of function calls, iteration keeps the control flow straightforward and typically consumes less memory, making it an optimal choice in scenarios where performance is critical. Understanding this difference is crucial in choosing the appropriate method for solving programming challenges.
The process of iteration is guided by a few core principles that ensure efficiency and effectiveness. Among these principles are:
- Initialization: Setting a starting point before entering the loop.
- Condition Checking: Determining whether to continue the loop based on a specific criterion.
- Increment/Decrement: Updating the loop variable to move closer to the stopping condition.
- Termination: Exiting the loop gracefully once the condition is met.
By adhering to these principles during the iterative process, developers can refine their understanding of the Difference Between Recursion and Iteration. While both techniques serve to carry out repetitive tasks, recognizing when to apply each leads to optimized and maintainable code structures. Furthermore, a clear grasp of iteration can often illuminate the limitations and potential overhead that may arise from a recursive approach.
Memory Usage: Recursion vs. Iteration
When evaluating the difference between recursion and iteration, memory usage is a crucial factor that often influences the choice between these two methodologies. Recursion typically consumes more memory because it relies on the function call stack. Each recursive call adds a new layer to the stack, which can quickly add up, especially for deep recursive calls. This can lead to significant memory utilization and, in some cases, stack overflow errors if the recursion depth exceeds the allocated stack space. On the other hand, iteration utilizes a fixed amount of memory regardless of the number of iterations, since it loops through a block of code without the overhead of additional calls.
Here’s a simplified comparison of memory usage:
Method | Memory Usage | Notes |
---|---|---|
Recursion | Higher | Each call adds to the stack, leading to potential overflow. |
Iteration | Lower | Fixed memory usage; generally more efficient for large datasets. |
understanding the memory implications of the difference between recursion and iteration can help developers make informed choices. For scenarios that involve deep recursion or large data sets, iteration is often the better choice due to its minimal memory footprint. The careful consideration of memory usage is essential for optimizing performance and preventing potential issues in software development.
Performance Considerations: Speed and Efficiency
When examining the difference between recursion and iteration, speed and efficiency often emerge as critical factors. Recursion involves a function calling itself to solve a problem, which can result in significant overhead due to the maintenance of multiple function calls in the call stack. This can lead to increased memory consumption and slower execution times, especially for deep recursive calls. In contrast, iteration uses loops to repeat actions and typically operates with a single function context, making it more memory efficient by avoiding the overhead associated with recursive call stacks.
To further illustrate the performance discrepancies, consider the following table that compares aspects of both methods:
Aspect | Recursion | Iteration |
---|---|---|
Memory Usage | Higher due to call stack | Lower; only uses loop variables |
Execution Speed | Slower for large problems | Generally faster for repetitive tasks |
Complexity | Simpler for divide-and-conquer | Clearer for straightforward loops |
Understanding the difference between recursion and iteration is essential for selecting the right approach for a given problem, especially when performance is a priority. As complexities increase, the iterative method often provides a more resource-efficient solution, while recursion can offer cleaner code in specific cases. Therefore, evaluating each scenario helps developers determine the most effective method to achieve their goals, balancing both speed and efficiency in their programming practices.
When to Choose Recursion Over Iteration
Choosing recursion over iteration is often influenced by the problem’s nature and the clarity it brings to the solution. Recursion can simplify the implementation of algorithms that deal with hierarchical structures or divide-and-conquer problems, such as tree traversals or calculating Fibonacci numbers. In scenarios where the task can be defined in terms of similar subtasks, recursion shines as a more intuitive approach. This method allows for elegant solutions by breaking down complex tasks into manageable pieces, illustrating the Difference Between Recursion and Iteration not only in functionality but also in the ease of understanding the code.
However, using recursion isn’t always the best choice. Performance can be significantly affected due to the overhead of function calls and the risk of stack overflow with deep recursion depths. This is where iteration often proves to be more efficient, especially for large datasets or when speed and memory usage are critical considerations. Thus, the decision on when to use recursion should also factor in aspects such as expected data size and comprehension of the Difference Between Recursion and Iteration, as well as the potential for optimizing the implementation with techniques like memoization. Choosing wisely allows for leveraging the strengths of both methods according to the specific requirements of the task at hand.
When Iteration Takes the Lead: Practical Scenarios
In situations where performance and memory usage are critical, iteration often outshines recursion. For example, when processing large datasets, an iterative approach can effectively manage memory consumption and enhance speed. This is particularly evident in scenarios such as:
- Data Processing: Iterative loops allow processing each item without the overhead of multiple function calls.
- Real-Time Systems: In environments where timing is crucial, minimizing function call stacks can be advantageous.
- Resource-Constrained Applications: Iteration minimizes the risk of stack overflow, which can occur with deep recursion.
Furthermore, iterative methods can simplify certain algorithm implementations, making them easier to read and maintain. A clear example lies in algorithms like sorting and searching, where the difference between recursion and iteration becomes pivotal in choosing an optimal solution. Consider this comparison in a table format:
Feature | Recursion | Iteration |
---|---|---|
Memory Usage | Higher due to call stack | Lower, uses loop variables |
Performance | Can be slower for large data | Generally faster |
Readability | Often more intuitive for complex problems | Can be straightforward |
Understanding the difference between recursion and iteration is crucial for developers aiming to write efficient code. Whether it’s managing resources effectively or enhancing performance, iteration plays a significant role in various practical scenarios.
Common Use Cases for Recursion
Recursion is a powerful programming technique that shines in solving problems that can be broken down into smaller, similar subproblems. One of the most common use cases is in tree traversal. When working with hierarchical data structures like trees, recursion allows for elegant solutions to traverse nodes. For example, in binary trees, recursive methods can efficiently navigate the structure through depth-first or breadth-first search algorithms. This showcases a notable difference between recursion and iteration, as recursion simplifies the code significantly while maintaining readability.
Another significant domain where recursion is advantageous is in the computation of factorials and generating Fibonacci sequences. These mathematical problems inherently rely on the previous elements to compute the next, making them perfect for recursive solutions. In contrast, iterative approaches may require more lines of code and can become less intuitive. Here’s a simple comparison of common problems and their recursive vs. iterative implementations:
Problem | Recursive Solution | Iterative Solution |
---|---|---|
Factorial | n! = n * factorial(n-1) | For loop to multiply n down to 1 |
Fibonacci | fib(n) = fib(n-1) + fib(n-2) | While loop maintaining last two values |
Tree Traversal | Visit node, recurse left, recurse right | Use a stack to manage nodes |
Common Use Cases for Iteration
Iteration is a fundamental technique utilized in programming across a multitude of applications. One of the most common use cases is within loops for automating repetitive tasks such as data processing and calculations. For instance, when performing large-scale data analysis, iterative algorithms efficiently traverse through datasets to derive insights, enabling functionalities like sorting, searching, and filtering data. In scenarios where performance is crucial, iteration may be preferred over recursion due to its reduced overhead, allowing faster execution, especially in resource-constrained environments.
Moreover, iteration shines in user interface design and enhancement. Tasks such as rendering lists or navigating through collections of data often leverage iterative constructs to cycle through elements smoothly. For example, a web application might utilize iteration to dynamically generate menu items based on user permissions, creating a responsive user experience. while exploring the difference between recursion and iteration, it’s evident that iteration provides a straightforward mechanism for efficiently handling repetitive tasks in a variety of programming scenarios.
The Impact on Code Readability and Maintainability
When exploring the difference between recursion and iteration, one of the primary considerations is how each approach affects code readability. Recursion, with its self-referential nature, often results in shorter and more elegant code. This can enhance clarity, especially for problems that have a natural recursive structure, like tree traversals or factorial calculations. The use of clear base cases and self-explanatory function names can make recursive solutions readily understandable. However, this elegance can come at the cost of complexity when the recursion depth increases or when readers are not familiar with the recursive design patterns.
In contrast, iterative solutions tend to be more explicit about their control flow, breaking down operations into discrete steps using loops. For those accustomed to traditional programming practices, this can enhance maintainability since it is often easier to trace the logic linearly. However, heavily nested loops can obfuscate the intention of the code, making it harder to read. Ultimately, the difference between recursion and iteration in terms of readability and maintainability hinges on the context of the problem and the familiarity of the team with both methods. This leads to considerations such as:
- Function Naming: Recursive calls should mirror the problem they solve for better understanding.
- Depth of Recursion: Too many recursive calls can lead to stack overflow, complicating maintainability.
- Loop Complexity: While iterations can be clearer, excessive nesting can hinder readability.
The following table summarizes some key aspects of recursion and iteration regarding their impact on maintainability:
Aspect | Recursion | Iteration |
---|---|---|
Readability | Often concise and elegant | More explicit but can be verbose |
Maintainability | Risk of stack overflow and harder for newcomers | Potentially clearer logic flow |
Performance | Can be slower due to overhead | Typically more efficient |
acknowledging the various impacts of the difference between recursion and iteration on code readability and maintainability can significantly shape programming choices. In practice, a well-informed developer needs to weigh the trade-offs presented by each methodology to optimize their code for both clarity and efficiency.
Debugging Recursion: Challenges and Strategies
When delving into the intricacies of the Difference Between Recursion and Iteration, one may encounter unique challenges, particularly in the realm of debugging recursion. Recursive functions, which call themselves with modified parameters, can lead to perplexing scenarios where determining the exact iteration can be complex. This results from the nested nature of recursive calls that can obscure the flow of execution. To effectively address these challenges, it’s crucial to leverage strategies such as:
- Utilizing print statements to trace the values and decisions made at each recursive call
- Employing debuggers that allow step-by-step execution, giving visibility into the recursive stack
- Developing base case checks early in the function to prevent runaway recursion
In contrast, understanding the iterative approach can simplify many debugging processes. Iteration relies on concrete loops, making tracking variable changes more straightforward. However, developers must remain vigilant about maintaining the correct state throughout the loop. A clear comprehension of the Difference Between Recursion and Iteration can enhance debugging efficacy. Here’s a brief comparison of their distinct characteristics:
Aspect | Recursion | Iteration |
---|---|---|
Structure | Self-calling functions | Loops (for, while) |
Memory Usage | Higher (stack memory) | Lower (single variable) |
Base Case | Essential for stopping | Not required |
Debugging Complexity | More complex | Easier |
Ultimately, mastering the Difference Between Recursion and Iteration not only enhances coding proficiency but also equips developers with the necessary tools to tackle debugging challenges acutely. Remaining aware of the specific attributes and considerations associated with each technique can foster more systematic and efficient problem-solving skills, especially in complex algorithmic tasks.
Best Practices for Implementing Recursion and Iteration
When approaching the difference between recursion and iteration, it’s essential to consider the specific use cases for each method. Recursion can be particularly powerful for solving problems that can be broken down into smaller, similar subproblems, such as traversing trees or processing complex data structures. To implement effective recursion, ensure that you:
- Define a clear base case: This prevents infinite loops and ensures termination of the recursive calls.
- Use concise and clear code: Keep your recursive function well-structured to enhance readability.
- Consider performance: Be aware of potential stack overflow issues and resource consumption.
In contrast, iteration is often favored for its simplicity and efficient use of memory when repeating actions or traversing linear data structures. Following best practices when implementing iteration is crucial; this includes:
- Using loops judiciously: Select the type of loop—such as for, while, or do-while—based on the scenario.
- Maintaining clarity: Ensure your loop conditions and increments/decrements are easy to understand.
- Managing loop efficiency: Optimize conditions to avoid unnecessary iterations and enhance performance.
Ultimately, understanding the difference between recursion and iteration will guide you in choosing the most suitable method for your programming challenges.
Frequently Asked Questions
Q&A: Exploring the Difference Between Recursion and Iteration
Q1: What is recursion, and how does it function?
A1: Recursion is a programming approach where a function calls itself in order to solve a problem. It typically involves two key components: a base case that terminates the recursion, and a recursive case that continues the process. For example, calculating the factorial of a number can be achieved through a recursive function that multiplies the number by the factorial of its predecessor until it reaches one.
Q2: What is iteration, and how does it differ from recursion?
A2: Iteration is a technique that repeats a set of instructions a defined number of times or until a specific condition is met. This is commonly implemented using loops, such as for
or while
loops. Unlike recursion, iteration does not involve the function calling itself; rather, it relies on a loop structure to cycle through the instructions, making it generally more memory-efficient.
Q3: Can you give an example of both recursion and iteration?
A3: Certainly! Let’s consider the task of calculating the Fibonacci sequence. In recursion, one might define a function that calls itself twice to find the sum of the two previous Fibonacci numbers. For iteration, a for
loop can sum the previous two numbers in a straightforward manner. While both methods yield the same result, the recursive approach can be more elegant and intuitive, while the iterative approach is often more efficient.
Q4: What are the advantages of using recursion?
A4: One of the major advantages of recursion is its simplicity in writing and reading code. It often leads to more elegant solutions, especially for problems that naturally fit recursive structures, such as tree traversals or solving complex combinatorial problems. Additionally, recursion can be easier to debug since each call is a separate instance that can be traced independently.
Q5: What are the drawbacks of recursion?
A5: The primary drawback of recursion is its potential for high memory consumption due to the call stack; every recursive call adds a new layer to the stack until the base case is reached. This can lead to stack overflow errors if the recursion goes too deep. Additionally, recursive solutions may become less efficient due to the overhead of function calls compared to iterative approaches.
Q6: When should I use recursion versus iteration?
A6: The choice between recursion and iteration often depends on the specific problem you are trying to solve. If the problem can be naturally divided into smaller subproblems or has a clear recursive structure, recursion may be the better choice. On the other hand, if memory efficiency and performance are critical, or if the solution involves simple repetition, iteration is often the safer bet.
Q7: Are there cases where a recursive solution can be converted to an iterative one?
A7: Absolutely! Many recursive solutions can be transformed into iterative ones by using data structures such as stacks or queues to manage the subproblems instead of relying on the call stack. This conversion can help mitigate high memory usage while still achieving the desired outcomes.
Q8: Is one method universally better than the other?
A8: Not necessarily. Both recursion and iteration have their unique strengths and weaknesses, and the choice largely depends on the context and requirements of the task. A skilled programmer understands the nuances of each technique and can select the appropriate one based on factors like code clarity, performance needs, and the complexity of the problem at hand.
Q9: Can concepts of recursion and iteration be combined?
A9: Yes, they can! Some algorithms might use both techniques within their structure. For instance, a recursive function could call an iterative process to handle certain parts of its data. This hybrid approach allows programmers to leverage the strengths of both recursion’s elegance and iteration’s efficiency.
Q10: What’s the takeaway for programmers when considering recursion and iteration?
A10: The essential takeaway is to choose the method that best suits the problem and context. Understanding both recursion and iteration enriches a programmer’s toolkit, enabling them to craft robust, efficient, and maintainable code. Recognize the strengths and weaknesses of each, and don’t hesitate to experiment with both to find the most elegant solution.
To Wrap It Up
understanding the difference between recursion and iteration is essential for any programmer. While both techniques serve to solve problems, they do so in fundamentally different ways. By grasping the difference between recursion and iteration, developers can choose the most efficient approach for their coding challenges.